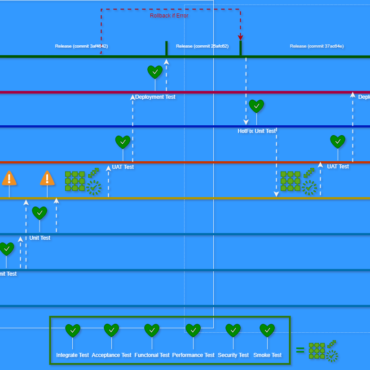
- help@firwl.com
- info@firwl.com
Data Security + Azure maurotommasi todayJanuary 15, 2024
Microsoft Azure offers a cloud service called Azure Key Vault that is intended to manage and safely store private data, including cryptographic keys, secrets, and certificates that are utilized by cloud services and apps. In addition to providing strong security features like access control, encryption, and auditing to protect sensitive data, it serves as a centralized repository. Secrets for application settings, certificates for communication security, and cryptographic keys for encryption and decryption are all managed and deployed more easily by developers and administrators with the aid of Azure Key Vault. This service offers a specialized, scalable solution for key and secret management in a cloud context, enhancing the security posture of Azure applications.
Table of Contents
Toggle
Using Azure Key Vault offers several benefits for secure and efficient management of sensitive information in a cloud environment:
Look here to get more specs about Azure Key Vault.
Azure Key Vault allows you to use Object Identifiers (OIDs) to uniquely identify various cryptographic objects stored in the vault, such as keys, secrets, and certificates. When you create these objects in Key Vault, they are assigned a unique OID, and you can use this identifier to manage and access the specific object.
Let’s take a look in Powershell, Python. and C++
# Powershell azureKeyVault.ps1
# Azure Key Vault configuration
$vaultName = "YourKeyVaultName"
$resourceGroup = "YourResourceGroupName"
# Connect to Azure Key Vault
Connect-AzAccount
$vault = Get-AzKeyVault -VaultName $vaultName -ResourceGroupName $resourceGroup
# Create a new key in Azure Key Vault
$keyName = "YourNewKey"
$key = Add-AzKeyVaultKey -VaultName $vaultName -Name $keyName -Destination 'software'
# Get the Object Identifier (OID) of the created key
$keyObjectId = (Get-AzKeyVaultKey -VaultName $vaultName -Name $keyName).Id
Write-Host "Key Object Identifier (OID): $keyObjectId"
For a testing environment, you can use Jupyter Notebook with Powershell Kernel. This allows you to create a markdown notebook to document the process and the results of your developed code. Here a guide on how to setup your VS Code to run Powershell in your Jupyter Notebook: https://powershellisfun.com/2022/08/04/jupyter-notebooks-in-vscode-with-powershell-support/
# Python azureKeyVault.py
from azure.identity import DefaultAzureCredential
from azure.keyvault.secrets import SecretClient
from azure.keyvault.keys import KeyClient
# Azure Key Vault configuration
vault_url = "https://YourKeyVaultName.vault.azure.net/"
# Authenticate to Azure Key Vault
credential = DefaultAzureCredential()
secret_client = SecretClient(vault_url=vault_url, credential=credential)
key_client = KeyClient(vault_url=vault_url, credential=credential)
# Create a new key in Azure Key Vault
key_name = "YourNewKey"
key = key_client.create_rsa_key(key_name)
# Get the Object Identifier (OID) of the created key
key_properties = key_client.get_key(key_name)
key_object_id = key_properties.id
print(f"Key Object Identifier (OID): {key_object_id}")
// C++ azureKeyVault.hpp
#include <iostream>
#include <azure/identity.hpp>
#include <azure/keyvault/secrets.hpp>
#include <azure/keyvault/keys.hpp>
// Azure Key Vault configuration
std::string vaultUrl = "https://YourKeyVaultName.vault.azure.net/";
// Authenticate to Azure Key Vault
auto credential = Azure::Identity::DefaultAzureCredential();
auto secretClient = Azure::KeyVault::Secrets::SecretClient(vaultUrl, credential);
auto keyClient = Azure::KeyVault::Keys::KeyClient(vaultUrl, credential);
// Create a new key in Azure Key Vault
std::string keyName = "YourNewKey";
auto key = keyClient.CreateRsaKey(keyName);
// Get the Object Identifier (OID) of the created key
auto keyProperties = keyClient.GetKey(keyName);
auto keyObjectId = keyProperties.Id;
std::cout << "Key Object Identifier (OID): " << keyObjectId << std::endl;
Azure Key Vault doesn’t typically expose Object Identifiers (OIDs) for Hardware Security Modules (HSMs) directly through its public APIs. However, you can interact with the Azure Key Vault HSM service using various SDKs and libraries provided by Azure. Below are examples in PowerShell, Python, and C++ for working with Azure Key Vault using the Azure SDK.
# Install Azure PowerShell Module
Install-Module -Name Az -Force -AllowClobber
# Sign in to your Azure account
Connect-AzAccount
# Set your Azure Key Vault details
$resourceGroupName = "YourResourceGroupName"
$keyVaultName = "YourKeyVaultName"
# Get the Key Vault details
$keyVault = Get-AzKeyVault -VaultName $keyVaultName -ResourceGroupName $resourceGroupName
# Display details, including the HSM information
$keyVault.Properties
from azure.identity import DefaultAzureCredential
from azure.keyvault.keys import KeyClient
# Set your Azure Key Vault details
vault_url = "https://YourKeyVaultName.vault.azure.net/"
# Authenticate to Azure Key Vault
credential = DefaultAzureCredential()
key_client = KeyClient(vault_url=vault_url, credential=credential)
# Get the Key Vault details, including HSM information
key_vault_properties = key_client.get_properties_of_key_vault()
print(key_vault_properties)
Be sure to install the required Python packages
pip install azure-identity azure-keyvault-keys
#include <iostream>
#include <azure/identity.hpp>
#include <azure/keyvault/keys.hpp>
// Set your Azure Key Vault details
std::string vaultUrl = "https://YourKeyVaultName.vault.azure.net/";
// Authenticate to Azure Key Vault
auto credential = Azure::Identity::DefaultAzureCredential();
auto keyClient = Azure::KeyVault::Keys::KeyClient(vaultUrl, credential);
// Get the Key Vault details, including HSM information
auto keyVaultProperties = keyClient.GetPropertiesOfKeyVault();
std::cout << keyVaultProperties << std::endl;
Remember that to get the Azure C++ components you need to:
sudo make install
Here an example of CMakeLists.txt file:
cmake_minimum_required(VERSION 3.10)
project(YourProject)
find_package(azure-storage-blobs REQUIRED)
add_executable(YourExecutable main.cpp)
target_link_libraries(YourExecutable PRIVATE Azure::azure-storage-blobs)
Cloud environment | DNS suffix for vaults | DNS suffix for managed HSMs |
---|---|---|
Azure Cloud | .vault.azure.net | .managedhsm.azure.net |
Microsoft Azure operated by 21Vianet Cloud | .vault.azure.cn | Not supported |
Azure US Government | .vault.usgovcloudapi.net | Not supported |
Azure German Cloud | .vault.microsoftazure.de | Not supported |
Object type | Identifier Suffix | Vaults | Managed HSM Pools |
---|---|---|---|
HSM-protected keys | /keys | Supported | Supported |
Software-protected keys | /keys | Supported | Not supported |
Secrets | /secrets | Supported | Not supported |
Certificates | /certificates | Supported | Not supported |
Storage account keys | /storage | Supported | Not supported |
Objects stored in Key Vault are versioned whenever a new instance of an object is created. Each version is assigned a unique object identifier. When an object is first created, it’s given a unique version identifier and marked as the current version of the object. Creation of a new instance with the same object name gives the new object a unique version identifier, causing it to become the current version.
Objects in Key Vault can be retrieved by specifying a version or by omitting the version to get the latest version of the object. Performing operations on objects requires providing a version to use specific version of the object.
Property | PowerShell | Python | C++ |
---|---|---|---|
Vault Name | $vaultName = (Get-AzKeyVault -VaultName 'YourVaultName').VaultName | vault_name = "<YourVaultName>" | std::string vaultName = "https://YourVaultName.vault.azure.net/"; |
Location | $location = (Get-AzKeyVault -VaultName 'YourVaultName').Location | Not directly available through Python SDK | Not directly available through C++ SDK |
Sku | $sku = (Get-AzKeyVault -VaultName 'YourVaultName').Sku.Name | Not directly available through Python SDK | Not directly available through C++ SDK |
Soft Delete Enabled | $softDeleteEnabled = (Get-AzKeyVault -VaultName 'YourVaultName').EnableSoftDelete | Not directly available through Python SDK | Not directly available through C++ SDK |
Purge Protection Enabled | $purgeProtectionEnabled = (Get-AzKeyVault -VaultName 'YourVaultName').EnablePurgeProtection | Not directly available through Python SDK | Not directly available through C++ SDK |
Access Policies | $accessPolicies = (Get-AzKeyVault -VaultName 'YourVaultName').AccessPolicies | access_policies = key_client.get_access_control() | auto accessPolicies = keyClient.GetAccessControl(); |
Network ACLs | Not directly available through PowerShell | network_acls = key_client.get_network_acls() | auto networkAcls = keyClient.GetPropertiesOfKeyVault(); |
Soft Delete Retention In Days | $softDeleteRetentionDays = (Get-AzKeyVault -VaultName 'YourVaultName').SoftDeleteRetentionInDays | Not directly available through Python SDK | Not directly available through C++ SDK |
Enabled For Deployment | $enabledForDeployment = (Get-AzKeyVault -VaultName 'YourVaultName').EnabledForDeployment | Not directly available through Python SDK | Not directly available through C++ SDK |
Enabled For Disk Encryption | $enabledForDiskEncryption = (Get-AzKeyVault -VaultName 'YourVaultName').EnabledForDiskEncryption | Not directly available through Python SDK | Not directly available through C++ SDK |
Enabled For Template Deployment | $enabledForTemplateDeployment = (Get-AzKeyVault -VaultName 'YourVaultName').EnabledForTemplateDeployment | Not directly available through Python SDK | Not directly available through C++ SDK |
Creation Date | $creationDate = (Get-AzKeyVault -VaultName 'YourVaultName').Created | creation_date = key_client.get_properties_of_key_vault().created | Not directly available through C++ SDK |
Tags | $tags = (Get-AzKeyVault -VaultName 'YourVaultName').Tags | Not directly available through Python SDK | Not directly available through C++ SDK |
Vault URI | $vaultUri = (Get-AzKeyVault -VaultName 'YourVaultName').VaultUri | vault_uri = key_client.vault_url | std::string vaultUri = "https://YourVaultName.vault.azure.net/"; |
Written by: maurotommasi
Coding maurotommasi
In the dynamic realm of programming languages, selecting the appropriate language for a given project is a pivotal decision. Developers must consider various factors, including readability, maintainability, and performance. One ...
Data Security maurotommasi
Coding maurotommasi
Copyright 2019 Cyber Security Design Concept by <a href="http://qantumthemes.com?rel=demo" target="_blank">QantumThemes</a>.
Post comments (0)