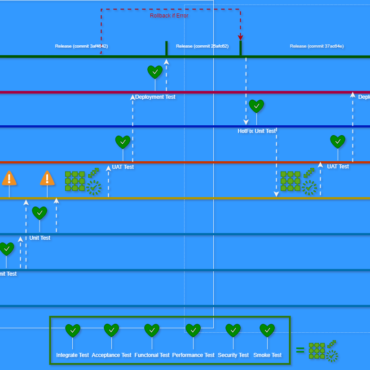
- help@firwl.com
- info@firwl.com
Let’s create a Python application that requires access to Azure Key Vault to fetch information from a Cosmos DB using a specific query, we can use the Azure SDK for Python. Below is a basic example using the azure-cosmos
library for Cosmos DB and the azure-identity
library for Azure Key Vault integration.
Table of Contents
Togglepip install azure-cosmos azure-identity
# Import necessary libraries
from azure.identity import DefaultAzureCredential
from azure.keyvault.secrets import SecretClient
from azure.cosmos import CosmosClient
# Function to retrieve a secret from Azure Key Vault
def get_secret_from_keyvault(vault_url, secret_name):
# Authenticate to Azure Key Vault using default credentials
credential = DefaultAzureCredential()
secret_client = SecretClient(vault_url=vault_url, credential=credential)
# Retrieve the secret value from Key Vault
secret_value = secret_client.get_secret(secret_name).value
return secret_value
# Function to query data from Cosmos DB
def query_cosmos_db(cosmos_connection_string, database_id, container_id, query):
# Connect to Cosmos DB using the provided connection string
cosmos_client = CosmosClient(cosmos_connection_string)
database_client = cosmos_client.get_database_client(database_id)
container_client = database_client.get_container_client(container_id)
# Execute the specified query and retrieve results
query_results = container_client.query_items(query, enable_cross_partition_query=True)
return list(query_results)
# Main function
if __name__ == "__main__":
# Azure Key Vault configuration
keyvault_url = "https://YourKeyVaultName.vault.azure.net/"
secret_name = "CosmosDBConnectionStringSecret"
# Cosmos DB configuration
cosmos_db_connection_string_secret = get_secret_from_keyvault(keyvault_url, secret_name)
cosmos_db_connection_string = cosmos_db_connection_string_secret.strip()
# Cosmos DB query
cosmos_db_database_id = "YourCosmosDBDatabaseId"
cosmos_db_container_id = "YourCosmosDBContainerId"
cosmos_db_query = "SELECT * FROM c WHERE c.YourProperty = 'YourValue'"
# Fetch data from Cosmos DB
results = query_cosmos_db(cosmos_db_connection_string, cosmos_db_database_id, cosmos_db_container_id, cosmos_db_query)
# Process and print the results
for result in results:
print(result)
The DefaultAzureCredential
is a part of the Azure Identity library in Python, which provides a default way to authenticate your application to Azure services. It simplifies the process of obtaining Azure Active Directory (AAD) tokens, which are required for authentication when interacting with Azure services, including Azure Key Vault.
Here’s how the DefaultAzureCredential
works:
DefaultAzureCredential
first checks for the presence of environment variables that might contain authentication information.AZURE_TENANT_ID
, AZURE_CLIENT_ID
, AZURE_CLIENT_SECRET
, and AZURE_USERNAME
.DefaultAzureCredential
attempts to use Managed Identity credentials if your code is running in an Azure environment that supports Managed Identity.DefaultAzureCredential
leverages the extension for authentication.DefaultAzureCredential
can use the Azure CLI’s credentials for authentication.DefaultAzureCredential
opens a browser window to interactively authenticate the user.DefaultAzureCredential
attempts to use MSI.By attempting various methods in sequence, the DefaultAzureCredential
ensures that it can acquire credentials in various environments and scenarios without requiring you to explicitly specify the authentication method.
For example, if your application is running in an Azure environment with Managed Identity, the DefaultAzureCredential
will automatically use that identity. If you’re developing locally and have signed in using the Azure CLI, it will use those credentials. The flexibility provided by DefaultAzureCredential
makes it suitable for a wide range of scenarios and environments.
The environment file .env can be used installing the package as follow: pip install python-env
.
We can set up our .env file as follow:
AZURE_TENANT_ID=your_tenant_id
AZURE_CLIENT_ID=your_client_id
AZURE_CLIENT_SECRET=your_client_secret
and use the .env configuration in the python code:
import os
from dotenv import load_dotenv
from azure.identity import DefaultAzureCredential
# Load environment variables from the .env file
load_dotenv()
# Use DefaultAzureCredential to authenticate
credential = DefaultAzureCredential()
This is an example code. It’s a good practice to standardize the code creating a class called, for example, azure-framework where inside we can split all functionalities based on their area (Azure Key Vult, Azure Cosmo DB and so on) and creating function to retrieve data as needed.
Written by: maurotommasi
Data Security maurotommasi
Microsoft Azure offers a cloud service called Azure Key Vault that is intended to manage and safely store private data, including cryptographic keys, secrets, and certificates that are utilized by ...
Data Security maurotommasi
Data Security maurotommasi
Copyright 2019 Cyber Security Design Concept by <a href="http://qantumthemes.com?rel=demo" target="_blank">QantumThemes</a>.
Post comments (0)