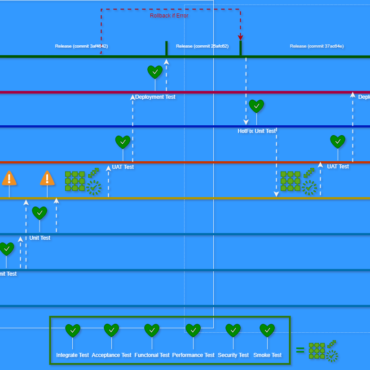
- help@firwl.com
- info@firwl.com
Coding + Data Security + Azure maurotommasi todayJanuary 15, 2024
Key Lifecycle Management (KLM) is a crucial aspect of cryptographic key management, ensuring the secure generation, distribution, usage, and retirement of cryptographic keys. In the context of Azure, a cloud computing platform by Microsoft, Key Vault provides a robust solution for managing cryptographic keys and secrets, offering advanced features for key lifecycle management.
Table of Contents
ToggleIn Azure Key Vault, keys can be generated using various algorithms and key sizes based on your security requirements.
# Powershell
$key = New-AzKeyVaultKey -VaultName 'YourKeyVault' -Name 'KeyName' -KeyType 'RSA' -KeySize 2048
# Python
key_client = KeyClient(vault_url='https://YourKeyVault.vault.azure.net/', credential=credential)
key = key_client.create_rsa_key('KeyName', size=2048)
Keys need to be securely stored to prevent unauthorized access. Azure Key Vault encrypts keys at rest and allows the configuration of access policies.
Set-AzKeyVaultAccessPolicy -VaultName 'YourKeyVault' -ResourceGroupName 'YourResourceGroup' -ObjectId 'YourUserOrAppObjectId' -PermissionsToKeys all
-VaultName 'YourKeyVault'
:
-ResourceGroupName 'YourResourceGroup'
:
-ObjectId 'YourUserOrAppObjectId'
:
-PermissionsToKeys all
:
all
).Parameter | Description |
---|---|
-VaultName | Name of the Azure Key Vault where the policy is applied. |
-ResourceGroupName | Name of the resource group containing the Key Vault. |
-ObjectId | Object ID of the user, group, or application. |
-PermissionsToKeys | Permissions granted for keys (e.g., ‘all’, ‘get’, ‘create’). |
Permissions Value | Description |
---|---|
all | Grants all permissions for keys. |
get | Grants permission to get keys. |
create | Grants permission to create keys. |
delete | Grants permission to delete keys. |
list | Grants permission to list keys. |
import | Grants permission to import keys. |
update | Grants permission to update keys. |
backup | Grants permission to backup keys. |
restore | Grants permission to restore keys. |
recover | Grants permission to recover keys. |
from azure.identity import DefaultAzureCredential
from azure.keyvault.secrets import SecretClient
# Key Vault configuration
vault_url = "https://YourKeyVaultName.vault.azure.net/"
resource_group = "YourResourceGroup"
object_id = "YourUserOrAppObjectId"
permissions_to_keys = ["all"]
# Authenticate to Azure Key Vault
credential = DefaultAzureCredential()
secret_client = SecretClient(vault_url=vault_url, credential=credential)
# Set Access Policy
secret_client.set_access_control(
{
"object_id": object_id,
"permissions": {"keys": permissions_to_keys}
}
)
vault_url = "https://YourKeyVaultName.vault.azure.net/":
resource_group = "YourResourceGroup"
:
object_id = "YourUserOrAppObjectId"
:
permissions_to_keys = ["all"]
:
all
).DefaultAzureCredential
is used to authenticate to Azure Key Vault using the application’s identity.set_access_control
method is used to set the access policy for the specified user, group, or application.object_id
and permissions
for keys.all
:The permissions granted by setting ["all"]
for keys in this example are similar to the PowerShell example:
get
create
delete
list
update
backup
restore
recover
purge
import
Keys are used for encryption, decryption, signing, and verification. Azure Key Vault allows applications to retrieve keys for cryptographic operations securely.
Here an example in Python: https://prodata.engineering/connect-a-python-app-to-azure-key-vault-and-cosmodb/
Regularly rotating keys enhances security. Azure Key Vault supports automated key rotation.
# Powershell
$key = Get-AzKeyVaultKey -VaultName 'YourKeyVault' -KeyName 'KeyName'
Update-AzKeyVaultKey -VaultName 'YourKeyVault' -ResourceGroupName 'YourResourceGroup' -KeyVersion $key.Key.Kid
# Python
key_client.rotate_key('KeyName')
Retiring or deleting keys is essential when they are no longer needed. Azure Key Vault supports soft-delete, allowing recovery within a retention period.
#Powershell
Remove-AzKeyVaultKey -VaultName 'YourKeyVault' -KeyName 'KeyName'
# Python
key_client.begin_delete_key('KeyName')
Key Vault provides comprehensive audit logs, enabling organizations to monitor key access and operations. For example we can use Aure Portal to monitor Key Valuts.
Operation | PowerShell | Python | C++ |
---|---|---|---|
Create Key | New-AzKeyVaultKey -VaultName 'YourKeyVault' -Name 'KeyName' -KeyType 'RSA' -KeySize 2048 | key_client.create_rsa_key('KeyName', size=2048) | auto keyClient = Azure::KeyVault::Keys::KeyClient(vaultUrl, credential); auto key = keyClient.CreateRsaKey('KeyName', 2048); |
Operation | PowerShell | Python | C++ |
---|---|---|---|
Set Access Policy | Set-AzKeyVaultAccessPolicy -VaultName 'YourKeyVault' -ResourceGroupName 'YourResourceGroup' -ObjectId 'YourUserOrAppObjectId' -PermissionsToKeys all | key_client.set_access_control(entry.acl) | keyClient.SetAccessPolicy('YourUserOrAppObjectId', KeyOperations::All); |
Operation | PowerShell | Python | C++ |
---|---|---|---|
Retrieve Key | $key = Get-AzKeyVaultKey -VaultName 'YourKeyVault' -KeyName 'KeyName' | key = key_client.get_key('KeyName') | auto key = keyClient.GetKey('KeyName'); |
Encrypt/Decrypt Data | $encryptedData = Encrypt-Data -Key $key -PlainText 'SensitiveData' | encrypted_data = key_client.encrypt('RSA1_5', plaintext) | auto result = cryptoClient.Encrypt(KeyId, EncryptParameters); |
Operation | PowerShell | Python | C++ |
---|---|---|---|
Get Key Version | $key = Get-AzKeyVaultKey -VaultName 'YourKeyVault' -KeyName 'KeyName' | key = key_client.get_key('KeyName') | auto key = keyClient.GetKey('KeyName'); |
Rotate Key | Update-AzKeyVaultKey -VaultName 'YourKeyVault' -ResourceGroupName 'YourResourceGroup' -KeyVersion $key.Key.Kid | key_client.rotate_key('KeyName') | auto result = keyClient.RotateKey('KeyName'); |
Operation | PowerShell | Python | C++ |
---|---|---|---|
Remove Key | Remove-AzKeyVaultKey -VaultName 'YourKeyVault' -KeyName 'KeyName' | key_client.begin_delete_key('KeyName') | keyClient.DeleteKey('KeyName'); |
Purge Key (Soft Delete) | Remove-AzKeyVaultKey -VaultName 'YourKeyVault' -KeyName 'KeyName' -InRemovedState -Purge | key_client.purge_deleted_key('KeyName') | keyClient.PurgeDeletedKey('KeyName'); |
Written by: maurotommasi
Python maurotommasi
Let’s create a Python application that requires access to Azure Key Vault to fetch information from a Cosmos DB using a specific query, we can use the Azure SDK for ...
Data Security maurotommasi
Coding maurotommasi
Copyright 2019 Cyber Security Design Concept by <a href="http://qantumthemes.com?rel=demo" target="_blank">QantumThemes</a>.
Post comments (0)