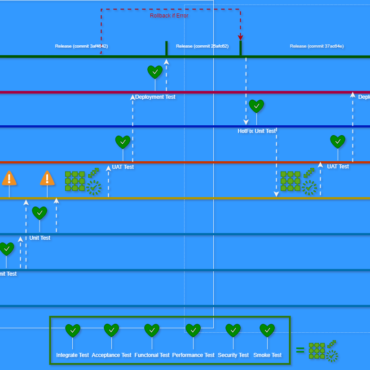
- help@firwl.com
- info@firwl.com
Coding + Basic Knowledge maurotommasi todayJanuary 8, 2024
In the dynamic realm of programming languages, selecting the appropriate language for a given project is a pivotal decision. Developers must consider various factors, including readability, maintainability, and performance. One key aspect in this decision-making process is understanding the differences in execution time across languages. In this article, we’ll explore examples in C++, JavaScript, Python, Java, and C# to showcase their respective execution times. Additionally, we’ll present a comprehensive table highlighting the pros and cons of each language.
Table of Contents
ToggleUnderstanding the performance of a programming language is crucial for developers and organizations alike. It directly impacts the efficiency and responsiveness of applications. A language’s execution speed influences tasks ranging from data processing to real-time systems. Knowledge of performance nuances aids in selecting the right language for specific use cases, ensuring optimal resource utilization. Additionally, insights into performance help developers write efficient code, identify bottlenecks, and optimize algorithms. Ultimately, this awareness empowers teams to make informed decisions, delivering high-performing software that meets user expectations and business requirements.
#include <iostream>
#include <chrono>
int main() {
const int size = 1000000;
int array[size];
// Initialize array with random values
for (int i = 0; i < size; ++i) {
array[i] = rand();
}
auto start = std::chrono::high_resolution_clock::now();
// Calculate the sum
long long sum = 0;
for (int i = 0; i < size; ++i) {
sum += array[i];
}
auto stop = std::chrono::high_resolution_clock::now();
auto duration = std::chrono::duration_cast<std::chrono::microseconds>(stop - start);
std::cout << "C++ Execution Time: " << duration.count() << " microseconds\n";
return 0;
}
JavaScript, often used for web development, is an interpreted language known for its flexibility. Here’s a JavaScript example using Node.js to find the sum of elements in an array:
const size = 1000000;
const array = new Array(size).fill(0).map(() => Math.random());
const start = new Date().getTime();
// Calculate the sum
const sum = array.reduce((acc, val) => acc + val, 0);
const stop = new Date().getTime();
const duration = stop - start;
console.log(`JavaScript Execution Time: ${duration} milliseconds`);
Python is recognized for its simplicity and readability. However, it tends to be slower than low-level languages. Here’s a Python example for finding the sum of elements in an array:
import time
import random
size = 1000000
array = [random.random() for _ in range(size)]
start = time.time()
# Calculate the sum
total_sum = sum(array)
stop = time.time()
duration = (stop - start) * 1000 # Convert to milliseconds
print(f"Python Execution Time: {duration} milliseconds")
Java, with its platform independence and robust ecosystem, strikes a balance between performance and ease of development. Here’s a Java example for finding the sum of elements in an array:
public class SumExample {
public static void main(String[] args) {
int size = 1000000;
double[] array = new double[size];
// Initialize array with random values
for (int i = 0; i < size; ++i) {
array[i] = Math.random();
}
long start = System.nanoTime();
// Calculate the sum
double sum = 0;
for (int i = 0; i < size; ++i) {
sum += array[i];
}
long stop = System.nanoTime();
long duration = (stop - start) / 1000000; // Convert to milliseconds
System.out.println("Java Execution Time: " + duration + " milliseconds");
}
}
C# is widely used for developing Windows applications and is part of the .NET framework. Here’s a C# example for finding the sum of elements in an array:
using System;
using System.Diagnostics;
class Program {
static void Main() {
int size = 1000000;
double[] array = new double[size];
// Initialize array with random values
for (int i = 0; i < size; ++i) {
array[i] = new Random().NextDouble();
}
Stopwatch stopwatch = new Stopwatch();
stopwatch.Start();
// Calculate the sum
double sum = 0;
for (int i = 0; i < size; ++i) {
sum += array[i];
}
stopwatch.Stop();
Console.WriteLine($"C# Execution Time: {stopwatch.ElapsedMilliseconds} milliseconds");
}
}
Now, let’s delve into a comprehensive table outlining the pros and cons of each language:
Language | Pros | Cons | Example Execution Time |
---|---|---|---|
C++ | – High performance, especially for resource-intensive tasks – Low-level memory control – Extensive standard library. | – Steeper learning curve – More verbose than higher-level languages. | ? |
JavaScript | – Versatility for web development – Asynchronous programming with event-driven architecture – Large ecosystem and community support. | – Single-threaded nature may limit performance in certain scenarios – Dynamic typing may lead to runtime errors. | ? |
Python | – Concise and readable syntax – Extensive standard library – Wide range of applications, from scripting to web development. | – Slower execution speed compared to compiled languages – Global Interpreter Lock (GIL) limits concurrent execution in multithreaded programs. | ? |
Java | – Platform independence through the Java Virtual Machine (JVM) – Strongly-typed language with strict object-oriented principles – Rich standard library and robust ecosystem. | – Requires the JVM, leading to slightly slower startup times – Verbosity compared to languages like Python and JavaScript. | ? |
C# | – Developed by Microsoft, well-suited for Windows applications – Strongly-typed language with a modern syntax – Integration with the .NET framework provides extensive libraries and tools. | – Tied to the Windows ecosystem, limiting cross-platform capabilities – Learning curve for certain advanced features and concepts. | ? |
Written by: maurotommasi
Coding maurotommasi
SOLID principles are a set of five design principles that aim to enhance the maintainability, flexibility, and scalability of object-oriented software. These principles were introduced by Robert C. Martin and ...
Copyright 2019 Cyber Security Design Concept by <a href="http://qantumthemes.com?rel=demo" target="_blank">QantumThemes</a>.
Post comments (0)